registerComponent
Adds your components to the Makeswift builder.
Arguments
-
componentComponentrequired
Your component to be registered. This can be a React component or a function.
-
optionsobjectrequired
Options for site version and locale.
Examples
Registering a box
This example shows how to register a Box
component. 'box'
is the value for type
, which must
be unique, as Makeswift uses this value to identify the component. This value shouldn’t change once you use the
component in the Makeswift builder. 'Box'
is the label
, which appears in
the Makeswift builder. The example applies a Style
control to the
className
prop.
import { Style } from "@makeswift/runtime/controls"
import { runtime } from './runtime'
function Box({ className }) {
return <div className={className}>I'm a box!</div>
}
runtime.registerComponent(Box, {
type: "box",
label: "Box",
props: {
className: Style({ properties: Style.All }),
},
})
Creating sections
This example shows how to register a Cube
and a Sphere
component under a “Visuals” section. In each
components’ label
, use slashes to separate the section name and component name. In the Makeswift
builder, both components appear under the same Visuals collapsible section:
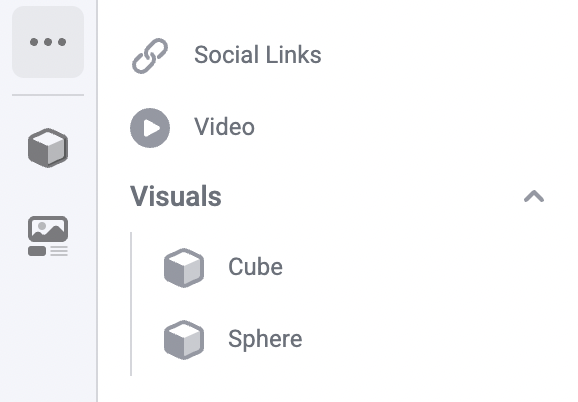
import { Style } from "@makeswift/runtime/controls"
function Cube({ className }) {
return <div className={className}>Cube!</div>
}
function Sphere({ className }) {
return <div className={className}>Sphere!</div>
}
runtime.registerComponent(Cube, {
type: "cube",
label: "Visuals/Cube",
props: {
className: Style({ properties: Style.All }),
},
})
runtime.registerComponent(Sphere, {
type: "sphere",
label: "Visuals/Sphere",
props: {
className: Style({ properties: Style.All }),
},
})
Adding custom icons
This example shows how to register an ImageGallery
component with a custom icon. We
import ComponentIcon
from @makeswift/runtime
and pass
ComponentIcon.Gallery
as the icon
for our component. In the builder
component tray, we can see our component with the selected icon.
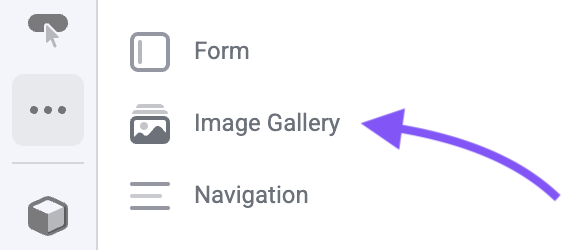
import { Style } from "@makeswift/runtime/controls"
import { ComponentIcon } from "@makeswift/runtime"
import { runtime } from './runtime'
function ImageGallery({ className }) {
return <div className={className}>I'm an image gallery!</div>
}
runtime.registerComponent(Cube, {
type: "image-galley",
label: "ImageGallery",
icon: ComponentIcon.Gallery,
props: {
className: Style({ properties: Style.All }),
},
})
Organizing registration code
As you register more components in makeswift/components.tsx
, you may notice this file growing rather large. To keep your codebase organized, we recommend breaking out your Makeswift registration code into separate files and co-locating it with your component file.
For example, if you have a Box
component, you can create a Box.makeswift.ts
file next to your Box.tsx
file. This file will contain the registration code for the Box
component.
Makeswift does not rely on this naming convention. Feel free to use whatever naming convention you prefer.
Then update your makeswift/components.tsx
file to import all of the component registrations, like so:
import "@/components/Box/Box.makeswift"
import "@/components/Cube/Cube.makeswift"
import "@/components/Sphere/Sphere.makeswift"
/*
* Add all of your component registrations here
*/
The file makeswift/components.ts
should be imported wherever you use <ReactRuntimeProvider>
in your app.
Was this page helpful?