Combobox
Adds a Combobox panel in the Makeswift builder to visually edit a generic prop from a list of options.
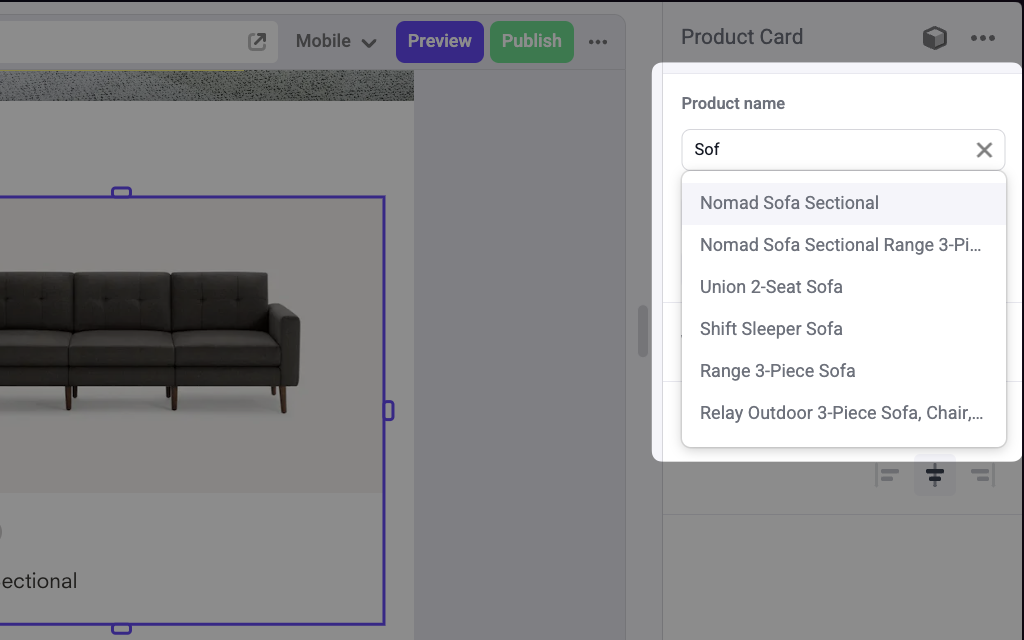
A Combobox panel on a Product Card component to pick a product by name
Params
Text for the panel label in the Makeswift builder.
A function that receives a query string and returns an array of type Option<T>
. This function may be async to fetch options asynchronously.
type getComboboxOptions<T> = (
query: string
) => ComboboxOption<T>[] | Promise<ComboboxOption<T>[]>
type ComboboxOption<T> = {
id: string
label: string
value: T
}
The label
field is displayed in the Makeswift builder and id
must be unique.
Prop type
The Combobox control passes the generic type T
from the selected Option
to your component, or undefined
if there is no value set in the builder.
Example
The following example adds a Combobox control to the product
prop of a Product Card component.
.makeswift.ts
is a naming convention for organizing Makeswift registration
code. Read more about this pattern
here.