Image
Adds an Image panel in the Makeswift builder to visually edit an image prop.
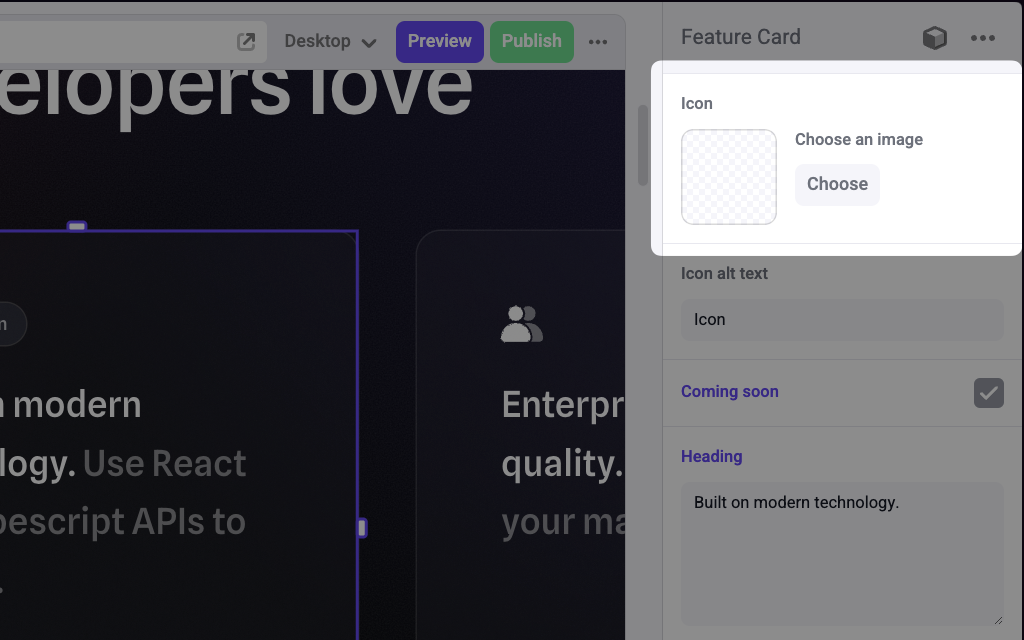
An image panel on a feature card component to pick an icon
Clicking “Choose” opens the Makeswift files manager, where you can upload an image or select an existing one from your media library.
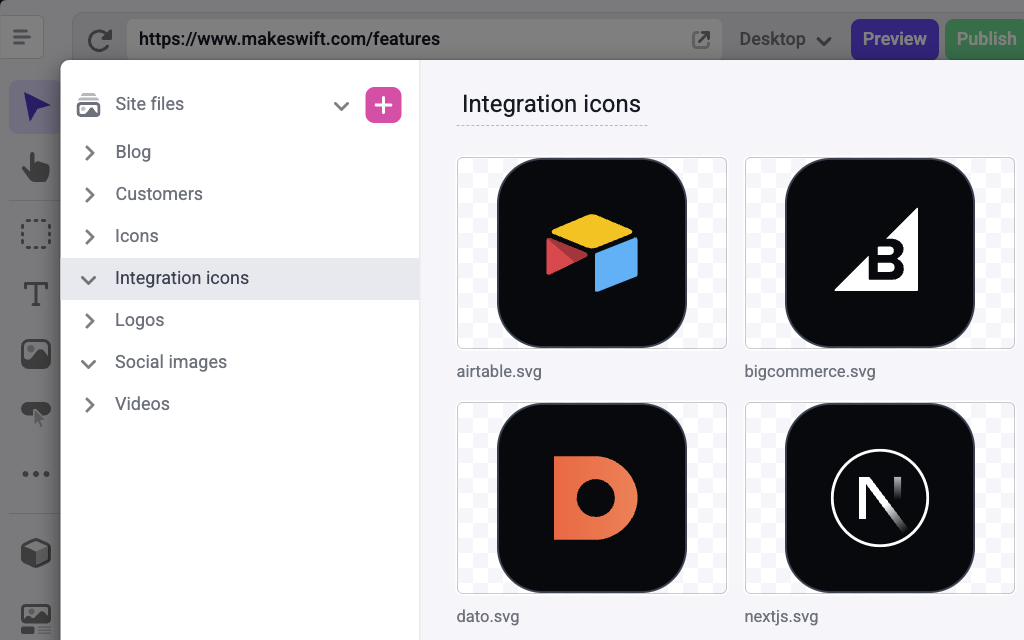
The Makeswift files manager
Params
Text for the panel label in the Makeswift builder.
Changes the prop type this component receives. If set to Image.Format.URL
, your component receives a string
value of the image url. If set to Image.Format.WithDimensions
, your component receives an object of type ImageWithDimensions
. This format is useful when using components like next/image
that require you to pass the image dimensions as props.
type ImageWithDimensions = {
url: string
dimensions: {
width: number
height: number
}
}
Prop type
If format
is set to Image.Format.URL
, the prop type is string
.
If format
is set to Image.Format.WithDimensions
, the prop type is ImageWithDimensions
.
Example
The following examples adds an Image control to the icon
prop of a Feature Card component.
Using Image.Format.URL
Using Image.Format.WithDimensions
.makeswift.ts
is a naming convention for organizing Makeswift registration
code. Read more about this pattern
here.